API¶
Generator API¶
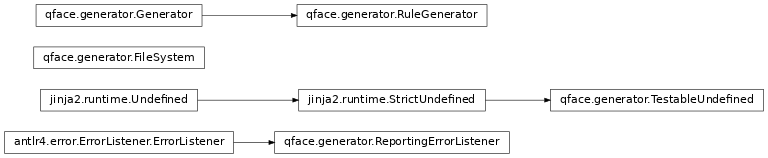
Generator Class¶
Provides an API for accessing the file system and controlling the generator
FileSystem Class¶
-
class
qface.generator.
FileSystem
[source]¶ Bases:
object
QFace helper functions to work with the file system
-
static
merge_annotations
(system, document)[source]¶ Read a YAML document and for each root symbol identifier updates the tag information of that symbol
-
static
parse
(input, identifier: str = None, use_cache=False, clear_cache=True, pattern='*.qface', profile=<EProfile.FULL: 'full'>)[source]¶ Input can be either a file or directory or a list of files or directory. A directory will be parsed recursively. The function returns the resulting system. Stores the result of the run in the domain cache named after the identifier.
Parameters: - path – directory to parse
- identifier – identifies the parse run. Used to name the cache
- clear_cache – clears the domain cache (defaults to true)
-
strict
= False¶ enables strict parsing
-
static
-
class
qface.generator.
Generator
(search_path, context={}, force=False)[source]¶ Bases:
object
Manages the templates and applies your context data
-
destination
¶ destination prefix for generator write
-
render
(name, context)[source]¶ Returns the rendered text from a single template file from the template loader using the given context data
-
source
¶ source prefix for template lookup
-
strict
= False¶ enables strict code generation
-
Template Domain API¶
This API is exposed to the Jinja template system.
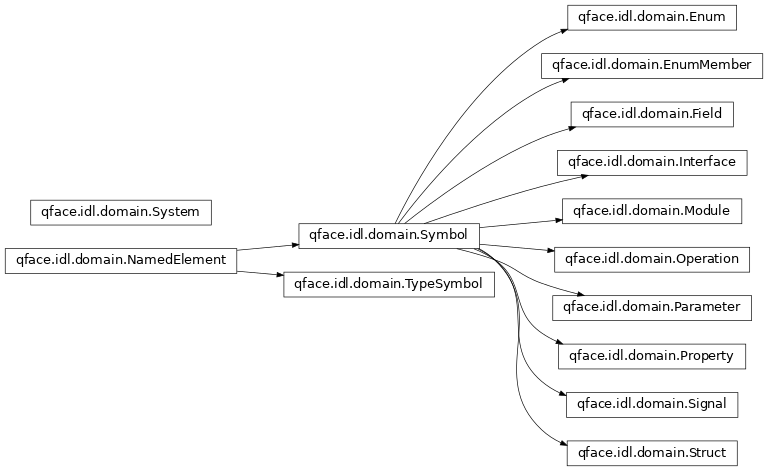
High Level Classes¶
-
class
qface.idl.domain.
System
[source]¶ Bases:
object
The root entity which consist of modules
-
modules
¶ returns ordered list of module symbols
-
-
class
qface.idl.domain.
Module
(name: str, system: qface.idl.domain.System)[source]¶ Bases:
qface.idl.domain.Symbol
Module is a namespace for types, e.g. interfaces, enums, structs
-
enums
¶ returns ordered list of enum symbols
-
imports
¶ returns ordered list of import symbols
-
interfaces
¶ returns ordered list of interface symbols
-
lookup
(name: str, fragment: str = None)[source]¶ lookup a symbol by name. If symbol is not local it will be looked up system wide
-
majorVersion
¶ returns the major version number of the version information
-
minorVersion
¶ returns the minor version number of the version information
-
module_name
¶ returns the last part of the module uri
-
name_parts
¶ return module name splitted by ‘.’ in parts
-
structs
¶ returns ordered list of struct symbols
-
Base Classes¶
-
class
qface.idl.domain.
NamedElement
(name, module: qface.idl.domain.Module)[source]¶ Bases:
object
-
module
= None¶ module the symbol belongs to
-
name
= None¶ symbol name
-
qualified_name
¶ return the fully qualified name (<module>.<name>)
-
-
class
qface.idl.domain.
Symbol
(name: str, module: qface.idl.domain.Module)[source]¶ Bases:
qface.idl.domain.NamedElement
A symbol represents a base class for names elements
-
comment
= None¶ comment which appeared in QFace right before symbol
-
contents
¶ return general list of symbol contents
-
kind
= None¶ the associated type information
-
system
¶ returns reference to system
-
-
class
qface.idl.domain.
TypeSymbol
(name: str, parent: qface.idl.domain.NamedElement)[source]¶ Bases:
qface.idl.domain.NamedElement
Defines a type in the system
-
is_bool
¶ checks if type is primitive and bool
-
is_complex
= None¶ if type represents a complex type
-
is_enum
¶ checks if type is an enumeration and reference is enum
-
is_enumeration
¶ checks if type is complex and instance of type Enum
-
is_flag
¶ checks if type is an enumeration and reference is flag
-
is_int
¶ checks if type is primitive and int
-
is_interface
¶ checks if type is interface
-
is_list
= None¶ if type represents a list of nested types
-
is_map
= None¶ if type represents a map of nested types. A key type is not defined
-
is_model
= None¶ if type represents a model of nested types
-
is_primitive
= None¶ if type represents a primitive type
-
is_real
¶ checks if type is primitive and real
-
is_string
¶ checks if type is primitive and string
-
is_struct
¶ checks if type is complex and struct
-
is_valid
¶ checks if type is a valid type
-
is_var
¶ checks if type is primitive and var
-
is_void
= None¶ if type represents the void type
-
nested
= None¶ nested type if symbol is list or model
-
parent
= None¶ the parent symbol of this type
-
reference
¶ returns the symbol reference of the type name
-
type
¶ return the type information. In this case: self
-